As someone whos fulltime job is as a software developer, there are always new things to learn, whether it be new design pattern, a new language or existing features in a language you've used for years.
That's where this came from, I had an inkling that delegates and events existed but never really investigated since my day job predominantly uses a different kind of event (not the one that's built into C#).
I'd been thinking for a while, that I wanted some kind of way to be able to make some kind of game that was more or less Quick Time Events and couple that with a game that revolved around the theatre. Which may or may not have been heavily inspired by the anime Shoujo Kageki Revue Starlight...
For the Quick Time Events, I'm certain there are better ways to do it but this is the way I've found would make things pretty extensible.
To test how viable this would be, I decided to implement a movement system where a level would have nodes where the player could automatically move from one location to another at the click of a button.
This movement system works by using a NavMeshSurface
and NavAgent
along with a selection of nodes to move too.
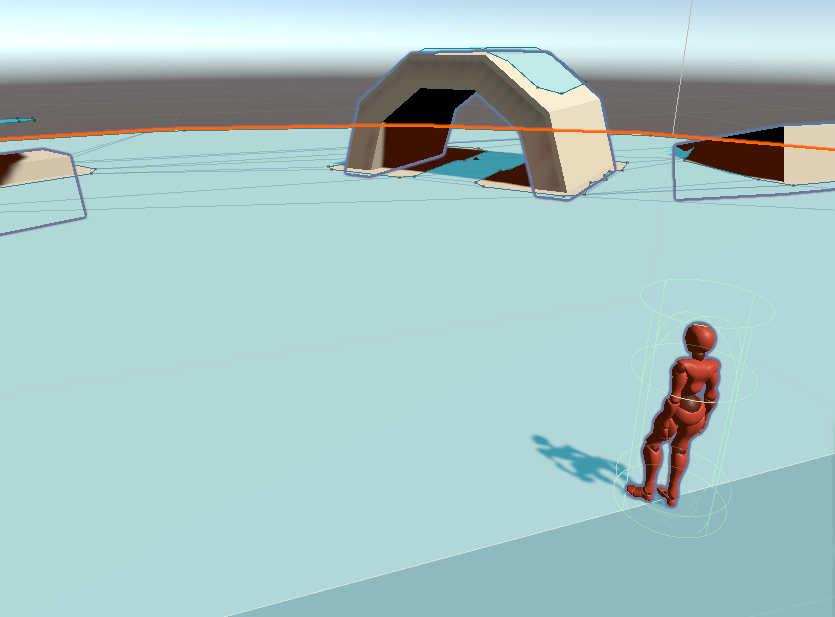
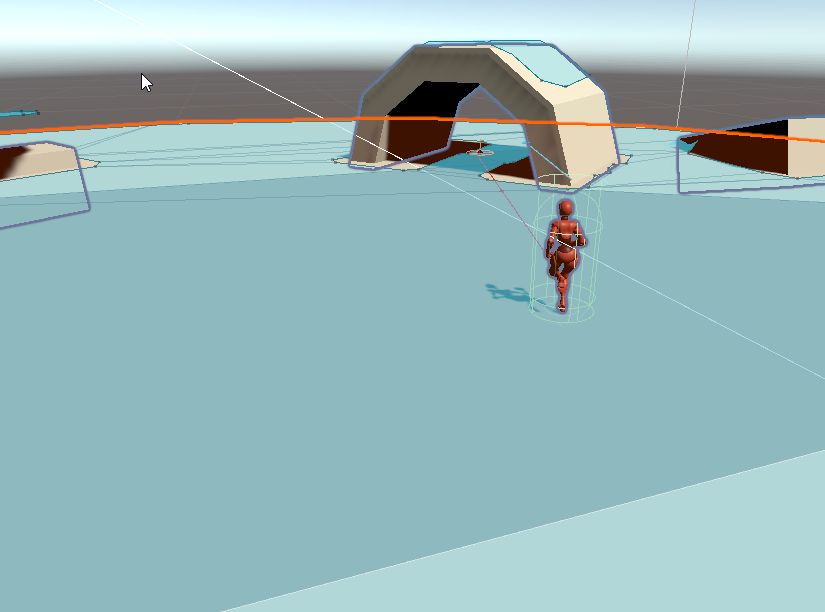
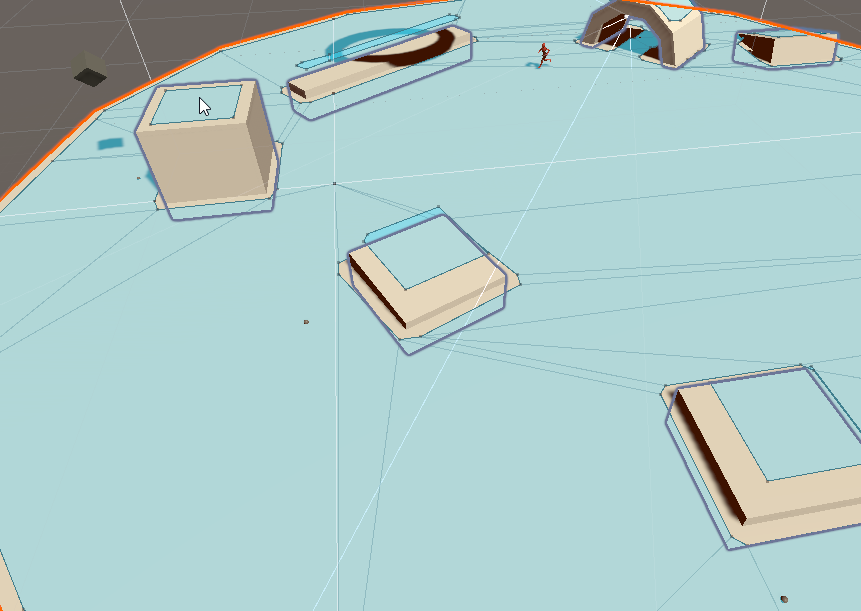
The main piece of code behind this is the following in the GameplayManagementSystem
class.
private delegate void PlayerMoveStartedEventHandler(object source, MoveToEventArgs args);
private event PlayerMoveStartedEventHandler PlayerMoveStarted;
private void Awake()
{
PlayerMoveStarted += _player.OnPlayerMoveStarted;
PlayerMoveStarted += _marker.OnPlayerMoveStarted;
}
These lines of code are what build the movement and the indication of where the player is going to end up.
Both the _player
and _marker
classes have a similar setup to each other where they have a public method called OnPlayerMoveStarted
which have the same parameters as the PlayerMoveStartedEventHandler
delegate above.
Side Note: The MoveToEventArgs
is a class that inherits from EventArgs
and holds the Vector3
to where the player will be going to.
The OnPlayerMoveStarted
method in both of the classes have their own way of moving to the required position.
_player
sets the new destination in the nav agent, which it automatically starts moving towards.
_marker
sets the destination in itself, resets the lerp timer and then starts lerping towards the location.
The main reason behind the difference in these is because I wanted the marker to not rely on the NavMeshSurface
and I wanted it to be very quick in moving to its new location, but not instantaneous.
This whole way of doing things is pretty extensible, especially if you have multiple things that take the structure of data but just use it in different ways.
A movement system is just one of those ways, there are many different things you could do with it. Maybe have it setup UI, audio cues, object spawning etc when a new controller is added for local multiplayer. In games with view interaction when streaming, have it listen for user commands and have them activated when a command is received.
I hope this has been informative and interesting. It was certainly interesting for me to write.